.Net Core Class - 1
E:\@udemy\@.Net Core\Ultimate ASP.NET Core 5 Web API Development Guide
https://github.com/trevoirwilliams/HotelListing-API-dotnet5/tree/ab2a4ce2a3209946297f4ed15de97b248bf27884
BASE - E:\@udemy\@.Net Core\[ FreeCourseWeb.com ] Udemy - RESTful API with ASP.NET Core Web API - Create and Consume\~Get Your Course Here !
RESUME -> 1 -7
NET framework has changed a lot over years it is now supporting Desktop based applications (WinForms), Web-based applications and windows universal platform (all windows platform tools from smartphone, tabs, hololens etc.) but still there are some limitations in .NET framework that it does not support cross-platform operating system like Linux and Mac (iOS).
Introduction to .NET Core
To overcome this limitation Microsoft has extend .NET framework capabilities and introduced .NET Core framework in Nov 2014. It is a free, open-source .NET framework that supports Linux and MacOS. It also contains CoreCLR and set of compiled libraries
What's the difference between .NET Core, .NET Framework
- Cross-Platform needs
- Best performant and scalable systems
- Command line style development for Mac, Linux or Windows
- NET core as an open source
- Easy to build cross-platform asp.net app on Windows, Mac, and Linux.
- It runs on .Net Core and Full .Net Framework.
- Asp.Net Core does not support WebForm. It supports MVC, Web API and Asp.Net Web pages originally added in .Net Core 2.0.
- Core did not support Web.config and Global.asax files. It is supporting appsettings.json.
- Core Browser refresh will compile and executed the code no need for re-compile.
- Ability to host on:
- A) Kestral
- B) IIS
- C) Apache
- E) Docker
Features of .NET Core
Yes, as it is the new generation .NET framework, it contains all features of .NET framework + its own new features, letās go through them
Cross platform support : .NET Core is supporting development of apps that can be deployed beyond windows platform, we can deploy our apps on Linux or on MacOS
Containers and Dockers support : Containers and dockers are very famous in these days, they can be easily adaptable by cloud technologies, Donāt worry .NET Core has full support of these components
Performance Improvement
MVC and web API merged : Before .NET Core, Developer needs to use MVC and Web API separately to create REST services using JSON, but .NET Core does job more simpler and merged them in a single module
Seamless support to Microservices
Command line development possible on Windows, MacOS, Linux
It support adjacent .NET versions per applications
.NET Core 2.1 Architecture
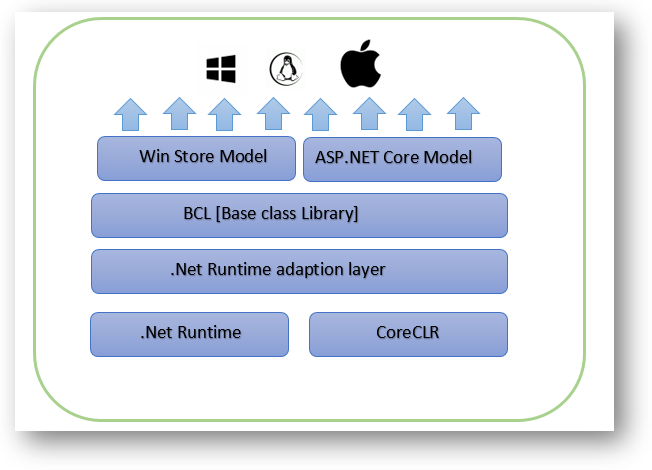
What is a Kestrel Web Server?
https://www.youtube.com/watch?v=3RpZGZDMd2A
As we already discussed the ASP.NET Core is a cross-platform framework. It means it supports developed and run applications on different types of operating systems such as Windows, Linux, or Mac.
The Kestrel is the cross-platform web server for the ASP.NET Core application. That means this Server supports all the platforms and versions that the ASP.NET Core supports. By default, it is included as the internal web server in the .NET Core application.
NET Core CLI
- When we run an ASP.NET Core application using the .NET Core CLI, then the .NET Core runtime uses Kestrel as the webserver
- The .NET Core CLI (Command Line Interface) is a cross-platform tool that is used for developing ASP.NET core applications on cross-platform such as windows, macs or Linux
ConfigureServices
method is a place where you can register your dependent classes with the built-in IoC container (ASP.NET Core refers dependent class as a Service). After registering the dependent class, it can be used anywhere in the application. You just need to include it in the parameter of the constructor of a class where you want to use it. The IoC container will inject it automatically.Configure
method is used to specify how the app responds to HTTP requests. The request pipeline is configured by adding middleware components to an IApplicationBuilder
instance. IApplicationBuilder
is available to the Configure
method, but it isnāt registered in the service container. Hosting creates an IApplicationBuilder
and passes it directly to the Configure
method.Index
method in the Home controller as specified below in the Configure
method:
Index
method in the HomeController.cs
returns the view Index.cshtml
which can be found under /Views/Home/ .

Inbuilt Dependency Injection (DI) support for ASP.NET Core
ASP.NET Core applications, dependency injection is inbuilt i.e. no setup headache for DI. Just create some services and get ready to use DI sample Core MVC application has DI inbuilt in it, letās open āStartup.csā and look forāConfigureServices(IServiceCollection services)ā method Its main purpose is the configuration of services like EF, Authentication, adding MVC handwritten custom services like IEmailServer and ISmsSender or custom service
What is Swagger?
- Swashbuckle.AspNetCore.Swagger: A Swagger object model expose SwaggerDocument objects in JSON.
- Swashbuckle.SwaggerGen : It provides the functionality to generate JSON Swagger.
- Swashbuckle.SwaggerUI : The Swagger UI tool uses the above documents for a rich customization for describing the Web API functionality.
Package installation
- Package Manager Console
NuGet Packages dialog
Swagger middleware
- services.AddSwaggerGen();
- app.UseSwagger();
- app.UseSwaggerUI(c =>
- {
- c.SwaggerEndpoint("/swagger/v1/swagger.json", "My Test1 Api v1");
- });

API infomation and description
- using Microsoft.OpenApi.Models;
- services.AddSwaggerGen(c => {
- c.SwaggerDoc("v1", new OpenApiInfo {
- Version = "v1",
- Title = "Test API",
- Description = "A simple example for swagger api information",
- TermsOfService = new Uri("https://example.com/terms"),
- Contact = new OpenApiContact {
- Name = "Your Name XYZ",
- Email = "xyz@gmail.com",
- Url = new Uri("https://example.com"),
- },
- License = new OpenApiLicense {
- Name = "Use under OpenApiLicense",
- Url = new Uri("https://example.com/license"),
- }
- });
- });

- https://localhost:5001/swagger/v1/swagger.json
Comments
Post a Comment