Linq -- Part 2
E:\@udemy\Projects\Linq
LINQ Aggregate Function
https://www.javatpoint.com/linq
Sorting Operators available in LINQ are:
- ORDER BY
- ORDER BY DESCENDING
- THEN BY
- THEN BY DESCENDING
- REVERSE
Operator | Description | Query Syntax |
---|---|---|
OrderBy | This operator will sort the values in ascending order. | ordeby |
OrderByDescending | This operator will sort the values in descending order. | Orderby ......descending |
ThenBy | This operator is used to perform the secondary sorting in ascending order. | Orderby...,.... |
ThenByDescending | This operator is used to perform the sorting in descending order. | Orderby....,....descending |
Reverse | This operator is used to reverse the order of elements in the collection. | Not applicable |
ThenBy
Syntax of LINQ ThenBy Operator
The Syntax of using the ThenBy operator in LINQ to implement the sorting on multiple fields is:
In the above Syntax, we are sorting the list of items using "Name," and we added another field "Roleid" by using the ThenBy condition to sort the list of items. Now, we will see this with the help of an example
LINQ Partition Operator
In LINQ, Partition Operators are used to partition the list/collections items into two parts and return one part of the list items. Here are the different types of partitioning operators available in LINQ.
- TAKE
- TAKEWHILE
- SKIP
- SKIPWHILE
By using these operators, we can partition the list/collection of items into two parts and return the one part of the list items.
The table shows more detailed information related to partition operators in LINQ.
Operator | Description | Query-Syntax |
---|---|---|
TAKE | This operator is used to return the specified number of elements in the sequence. | Take |
TAKEWHILE | This operator is used to return the elements in the sequence which satisfy the specific condition. | Takewhile |
SKIP | This operator is used to skip the specified number of elements in a sequence and return the remaining elements. | Skip |
SKIPWHILE | This operator is used to skip the elements in a sequence based on the condition, which is defined as true. | Skipwhile |
Syntax of LINQ Take Operator
The Syntax of LINQ Take operator is to return the specified number of elements from the list/collection.
- string[] countries = { "India", "USA", "Russia", "China", "Australia", "Argentina" };
- //here take() method will return the value from the String array upto three numbers.
- IEnumerable<string> result = countries.Take(3);
- foreach (string s in result)
- {
- Console.WriteLine(s);
- }
LINQ ToLookup() Method
ToLookup operator in LINQ is an extension method, and it is used to extract a set of key/value pairs from the source. Here, each element in the resultant collection is a generic Lookup object. Lookup object holds the Key and subsequence items that matched with the Key.
C# Code
In the above syntax, we are converting the collection of "objEmployee" to key/value pair list using the ToLookup operator.
LINQ Cast() Method
In LINQ, Cast operator is used to cast/convert all the elements present in a collection into a specified data type of new collection. In case if we try to cast/convert different types of elements (string/integer) in the collection, then the conversion will fail, and it will throw an exception.
Syntax of LINQ Cast () conversion operator
C# Code
LINQ OfType() Method
OfType() operator is used to return the element of the specific type, and another element will be ignored from the list/collection.
Syntax of LINQ OfType() Operator
The syntax of using the OfType () LINQ Operator is to get the specified type of elements from the list/collection is:
C# Code
In the above syntax, we are trying to get only the string elements from the collection of "obj" by using the OfType operator
- //Create an object of ArrayList and add the values
- ArrayList obj = new ArrayList();
- obj.Add("Australia");
- obj.Add("India");
- obj.Add("UK");
- obj.Add("USA");
- obj.Add(1);
- //ofType() method will return the value only the specific type
- IEnumerable<string> result = obj.OfType<string>();
- //foreach loop is applied to print the value of the item
- foreach (var item in result)
- {
- Console.WriteLine(item);
- }
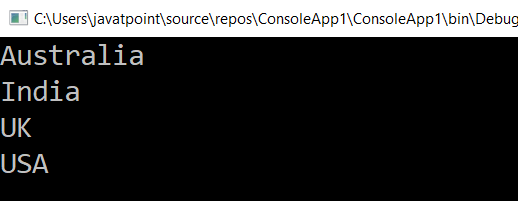
LINQ AsEnumrable() Method
In LINQ, AsEnumerble() method is used to convert the specific type of given list to its IEnumerable() equivalent type.
Syntax of LINQ AsEnumerable() Method
C# Code
In the above syntax, we are converting the list of "numarray" to IEnumerable type.
- int[] NumArray = new int[] { 1, 2, 3, 4,5};
- //After applying the AsEnumerable method the output will be store in variable result
- var result = NumArray.AsEnumerable();
- //Now we will print the value of variable result one by one with the help of foreach loop
- foreach (var number in result)
- {
- Console.WriteLine(number);
- }
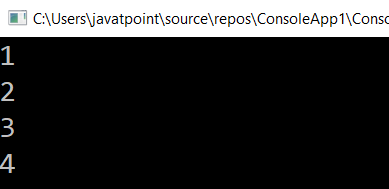
LINQ ToDictionary() Method
In LINQ, ToDictionary() Method is used to convert the items of list/collection(IEnumerable<T>) to new dictionary object (Dictionary<TKey,TValue>) and it will optimize the list/collection items by required values only.
Syntax of LINQ ToDictionary Method
Here is the syntax of using the LINQ ToDictionary() operator.
C# Code
- List<Student> objStudent = new List<Student>()
- {
- new Student() { Id=1,Name = "Vinay Tyagi", Gender = "Male",Location="Chennai" },
- new Student() { Id=2,Name = "Vaishali Tyagi", Gender = "Female", Location="Chennai" },
- new Student() { Id=3,Name = "Montu Tyagi", Gender = "Male",Location="Bangalore" },
- new Student() { Id=4,Name = "Akshay Tyagi", Gender = "Male", Location ="Vizag"},
- new Student() { Id=5,Name = "Arpita Rai", Gender = "Male", Location="Nagpur"}
- };
- /*here with the help of ToDictionary() method we are converting the colection
- of information in the form of dictionary and will fetch only the required information*/
- var student = objStudent.ToDictionary(x => x.Id, x => x.Name);
- //foreach loop is used to print the information of the student
- foreach (var stud in student)
- {
- Console.WriteLine(stud.Key + "\t" + stud.Value);
- }
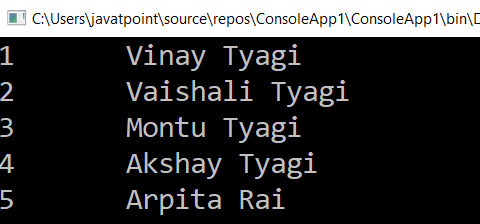
LINQ Element Operators
In LINQ, element operators are used to returning the first and last element of the list or single element from the collection or a specific element based on the index value from the collection.
In LINQ, we have different types of element operators available. These are:
- First
- FirstOrDefault
- Last
- LastOrDefault
- ElementAt
- ElementAtOrDefault
- Single
- SingleOrDefualt
- DefaultfEmpty
Operators | Description |
---|---|
First | It returns the first element in sequence or from the collection based on the condition. |
FirstOrDefault | It is the same as First, but it returns the default value in case when no element is found in the collection. |
Last | It returns the last element in sequence or the last element based on the matching criteria. |
ElementAt | It returns an element from the list based on the specific index position. |
ElementAtOrDefault | It is the same as ElementAt, but it returns the default value in case if no element is present at the specified index of the collection. |
Single | It returns the single specific element from the collection. |
SingleOrDefault | It is the same as Single, but it returns the default value in case if no element is found in the collection. |
DefaultfEmpty | It returns the default value in case if the list or collection contains empty or null values. |
LINQ First() Element
In LINQ, the First () method/operator is used to return the first element from the sequence of the items in the list or collection or the first element in the sequence of items in the list based on the specified condition. In case if no element is present in the list/collection based on the specified condition, then LINQ FIRST () method will throw an error.
Syntax of LINQ FIRST () method
The syntax of the first method to get the first element from the list is:
From the above syntax, we are getting the first element from the "objList" collection using the LINQ FIRST () method.
- int[] objList = { 1, 2, 3, 4, 5 };
- //First() method is used to return the first element from the array 'objList'
- int result = objList.First();
- Console.WriteLine("Element from the List: {0}", result);

LINQ FirstOrDefault() Method
In LINQ, FirstOrDefault() Operator is used to return the first element from the list/collection. FirstOrDefault() Operator is same as LINQ First() Operator and the only difference is, in case if the lists returns no elements then LINQ FirstOrDefault operator method will return default value.
Syntax of FirstOrDefault Method
Here is the syntax of the LINQ FirstOrDefault operator to return the first element from the list or in case if lists return no element.
From the above syntax, we are trying to get the First element or default element from the "objList" collection by using LINQ FirstOrDefault() operator.
LINQ Last() Method
The last () Method in LINQ is used to return the last element from the list/collection. LINQ Last() method will throw an exception in case if the list/collection returns no elements.
Syntax of LINQ Last() method
LINQ ElementAt() Method
In LINQ, ElementAt() operator is used to return the elements from the list/collection based on the specified index.
Generally, in the list, the index will start with zero, so if we want to get the first element, then there is a need to send zero (0) as an index position.
Syntax of LINQ ElementAt() Method
LINQ ElementAtOrDefault() Method
In LINQ, ElementAtOrDefault() method is used to get the element at specified index position in the list/collection and it is same as LINQ ElementAt() method. The only difference between the both ElementAtOrDefault() and ElementAt() is that it will return default value only. On the other hand, if the specified index position of the element does not exist in the list, then in that case also this will return the default value.
Syntax of LINQ ElementAtOrDefault() Method
The syntax of using the LINQ ElementAtOrDefault() to get the element at specified index position.
LINQ Single() Method
In LINQ, the Single() method is used to return the single element from the collection, which satisfies the condition. In case, if the Single() method found more than one element in collection or no element in the collection, then it will throw the "InvalidOperationException" error.
Syntax of LINQ Single () Method
The syntax of using the LINQ Single () method to get a single element from the collection.
- List<Student> objStudent = new List<Student>()
- {
- new Student() { Name = "Shubham Rastogi", Gender = "Male",Location="Chennai" },
- new Student() { Name = "Rohini Tyagi", Gender = "Female", Location="Chennai" },
- new Student() { Name = "Praveen Alavala", Gender = "Male",Location="Bangalore" },
- new Student() { Name = "Sateesh Rastogi", Gender = "Male", Location ="Vizag"},
- new Student() { Name = "Madhav Sai", Gender = "Male", Location="Nagpur"}
- };
- //initialize the array objList
- int[] objList = { 1 };
- //objStudent.Single() used to select the student
- var user = objStudent.Single(s => s.Name == "Shubham Rastogi");
- string result = user.Name;
- int val = objList.Single();
- Console.WriteLine("Element from objStudent: {0}", result);
- Console.WriteLine("Element from objList: {0}", val);
LINQ SingleOrDefault Method
In LINQ, SingleOrDefault() method is used to return the single element. In case if there are no elements present in the list/collection, then this will return more than one element and will throw an exception like a Single () method.
Syntax of LINQ SingleOrDefault() Method
Here is the syntax of using the LINQ SingleOrDefault() method to get the single element from the collection.
If the number object has more than one value it'll throw an error
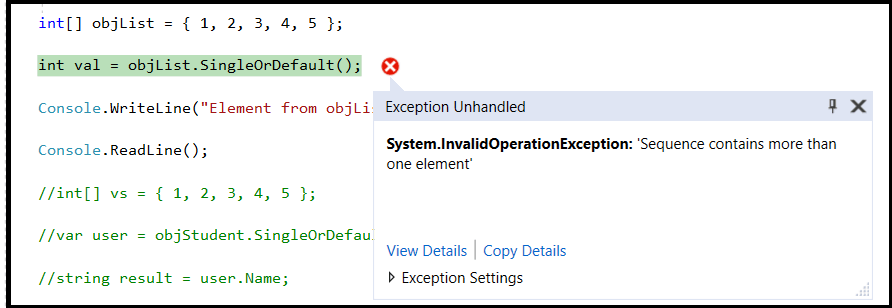
LINQ DefaultfEmpty() Method
In LINQ, DefaultfEmpty() method is used to return the default value in that case if the list/collection contains the null or empty value; otherwise, it will return the element from the sequence in the collection.
Syntax of using the LINQ DefaultfEmpty() method to get the list of the elements when the list returns an empty or null value.
- //create an array 'b'
- int[] b = { };
- int[] a = { 1, 2, 3, 4, 5 };
- //with the help of DefaultfEmpty try to fetch the value from both of the list
- var result = a.DefaultIfEmpty();
- var result1 = b.DefaultIfEmpty();
- Console.WriteLine("----List1 with Values----");
- //with the help of foreach loop we will print the value of 'result' variable
- foreach (var val1 in result)
- {
- Console.WriteLine(val1);
- }
- Console.WriteLine("---List2 without Values---");
- //with the help of foreach loop we will print the value of 'result1' variable
- foreach (var val2 in result1)
- {
- Console.WriteLine(val2);
- }
Output:
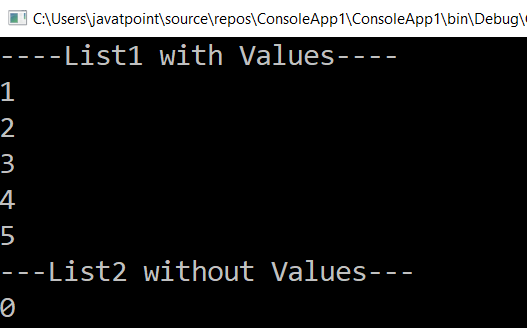
Comments
Post a Comment