Linq - Basics - Part 1
E:\@udemy\Projects\Linq
* LinQ is a 'Language Integrated Query
* LINQ provides the new way to manipulate the data, whether it is to or from the database or with an XML file or with a simple list of dynamic data. LINQ is a uniform query system in C# to retrieve the data from different sources of data and formats
* LINQ is built in C#. It is used to retrieve the data from different types of sources such as XML, docs, collections, ADO.Net DataSet, Web Service, MS SQL Server, and other databases server.
LINQ has three ways to write the queries:
- Using Query Syntax
- Using Method Syntax
- Using Mixed Syntax
Many times we use a combination of both. Now, with the evolution of the entity framework, the importance of LINQ has grown much higher as compared to old times.
have different types of LINQ Objects available in C# and VB.NET.
- LINQ To Objects
- LINQ To DataSets
- LINQ To SQL
- LINQ to XML
- LINQ To Entities
Advantages of LINQ
In our applications, the benefits of LINQ are:
- We do not need to learn new query language syntaxes for different sources of data because it provides the standard query syntax for the various data sources.
- In LINQ, we have to write the Less code in comparison to the traditional approach. With the use of LINQ, we can minimize the code.
- LINQ provides the compile-time error checking as well as intelligence support in Visual Studio. This powerful feature helps us to avoid run-time errors.
- LINQ provides a lot of built-in methods that we can be used to perform the different operations such as filtering, ordering, grouping, etc. which makes our work easy.
- The query of LINQ can be reused.
Disadvantages of LINQ
Disadvantages of LINQ are:
- With the use of LINQ, it's very difficult to write a complex query like SQL.
- It was written in the code, and we cannot make use of the Cache Execution plan, which is the SQL feature as we do in the stored procedure.
- If the query is not written correctly, then the performance will be degraded.
- If we make some changes to our queries, then we need to recompile the application and need to redeploy the dll to the server.
LINQ Query Syntax
LINQ is one of the easiest ways to write the complex LINQ Queries in an accessible and readable format. The syntax of this type of Query is much similar to SQL queries.
Syntax of LINQ is as:
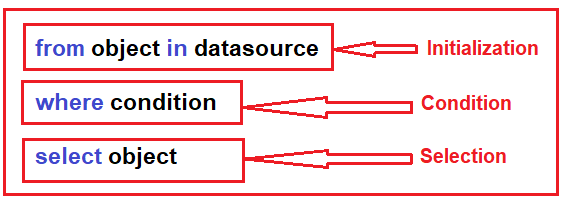
LINQ Query Syntax in C#
int[] Num = { 1, 2, 3, 4, 5, 6, 7, 8, 9 };
IEnumerable<int> result = from numbers in Num
where numbers >3
select numbers;
LINQ Method Syntax
Method Syntax becomes the most popular nowadays to write LINQ queries. It uses a lambda expression to define the condition for the Query. Method Syntax is easy to write simple queries to perform the read-write operations on a particular data source. For the complex queries, Method Syntaxes are a little hard as compared to the Query Syntax.
- List<int>integerList = new List<int>()
- {
- 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
- };
- //LINQ Query using Method Syntax
- varMethodSyntax = integerList.Where(obj =>obj> 5).ToList();
- //Execution
- foreach (var item inMethodSyntax)
- {
- Console.Write(item + " ");
- }
LINQ Mixed Syntax
LINQ Mixed Syntax is a combination of both Query and MethodSyntax.
- List<int>integerList = new List<int>()
- {
- 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
- };
- //LINQ Query using Mixed Syntax
- varMethodSyntax = (fromobjinintegerList
- whereobj> 5
- selectobj).Sum();
- //Execution
- Console.Write("Sum Is : " + MethodSyntax);
This whole syntax forms a Lambda Expression.
Here, we are taking an example of commonly used Expression:
X=>x+10
- //list to store the countries type of string
- List<string> countries = new List<string>();
- countries.Add("India");
- countries.Add("US");
- countries.Add("Australia");
- countries.Add("Russia");
- //use lambda expression to show the list of the countries
- IEnumerable<string> result = countries.Select(x => x);
- //foreach loop to display the countries
- foreach (var item in the result)
- {
- Console.WriteLine(item);
- }
Comments
Post a Comment