Linq - part 3
LINQ Join() Operators
In LINQ, Join() operators are used to join the two or more lists/collections and get the matched data from the collection based on the specified conditions. The behavior and functionality of Join() operators are the same as SQL joins.
In LINQ, We have different types of joins available. These are:
- INNER JOIN
- LEFT OUTER JOIN
- CROSS JOIN
- GROUP JOIN
Operator | Description |
---|---|
Inner Join | It returns the element from the collections which satisfy the specified expression. |
Left Outer Join | It returns all the elements from the left side collection and matching the elements with the right side collection. |
Cross Join | It returns the Cartesian product of elements in collections. |
Group Join | The join clause with an 'into' expression is called a group join |
LINQ Inner Join
In LINQ, Inner join is used to return only the matched records or elements from the collections based on the specified conditions.
Syntax of LINQ Inner Join
Here is the syntax of using the LINQ Inner join to get the elements from the collections based on the specified condition.
- List<Department> Dept = new List<Department>
- (){
- new Department{DepId=1,DepName="Software"},
- new Department{DepId=2,DepName="Finance"},
- new Department{DepId=3,DepName="Health"}
- };
- List<Employee>Emp = new List<Employee>
- ()
- {
- new Employee { EmpId=1,Name = "Akshay Tyagi", DeptId=1 },
- new Employee { EmpId=2,Name = "Vaishali Tyagi", DeptId=1 },
- new Employee { EmpId=3,Name = "Arpita Rai", DeptId=2 },
- new Employee { EmpId=4,Name = "Sateesh Alavala", DeptId =2},
- new Employee { EmpId=5,Name = "Madhav Sai"}
- };
- var result = from d in Dept
- join e in Emp
- on d.DepId equals e.DeptId
- select new
- {
- EmployeeName = e.Name,
- DepartmentName = d.DepName
- };
- foreach (var item in result)
- {
- Console.WriteLine(item.EmployeeName + "\t | " + item.DepartmentName);
- }
LINQ Left Outer Join
In LINQ, LEFT JOIN or LEFT OUTER JOIN is used to return all the records or elements from the left side collection and matching the elements from the right side of the collection.
In LINQ, to achieve the LEFT Join behavior, it is mandatory to use the "INTO" keyword and "DefaultfEmpty()" method.
Syntax of LINQ Left Outer Join
The syntax of using the LINQ Left Outer Join to get all the elements from the collection and matching the element from the right collection.
LINQ Cross Join
In LINQ, Cross join will produce the Cartesian product of the collections of items. There is no need for any condition to join the collection.
In LINQ Cross join, each element on the left side collection will be mapped to all the elements on the right side collection.
Syntax of LINQ Cross Join
Here is the syntax of using the LINQ Cross join to get the Cartesian product of the collection items.
LINQ Group Join
In LINQ, a Join clause with an 'into' expression is called a Group join. In LINQ, Group join produces a sequence of the elements of the objects, which is based on the matching element from both left and right collections.
In case, if no matching element found from the right collection with the left collection, then the join clause will return an empty array. In LINQ, Group join is an equivalent to inner equi join except for the result of the elements organized into groups.
Syntax of LINQ Group Join
The syntax of using the LINQ Group Join to get the matching element from the given collections based on our requirement.
From the above syntax, we used a Join clause with an 'into' expression to get the matching elements from the collections.
LINQ Set Operations
In LINQ, Set Operators are used to return the set of the result based on the presence or absence of equivalent elements within the same or separate collections.
In LINQ, we have different types of set operators available. These are:
- UNION
- INTERSECT
- DISTINCT
- EXCEPT
Operators | Description |
---|---|
UNION | Union operator combines multiple collections into single collection and return the resultant collection with unique element. |
INTERSECT | It returns the element in a sequence, which is common in both the input sequence. |
DISTINCT | It removes the duplicate elements from the collection and returns the collection with unique values. |
EXCEPT | It returns the sequence element from the first input sequence, which is not present in the second input sequence. |
LINQ Union Method
In LINQ, the Union method or operator is used to combine the multiple collections into a single collection and return the resultant collection with a unique element.
Syntax of LINQ union Method
Here is the syntax of using the union method to get the unique elements from the multiple collections.
In the above syntax, we are combining two collections to get the result as a single collection using the union method.
- string[] count1 = { "India", "USA", "UK", "Australia" };
- string[] count2 = { "India", "Canada", "UK", "China" };
- //count1.Union(count2) is used to find out the unique element from both the collection
- var result = count1.Union(count2);
LINQ Intersect Method
In LINQ, the Intersect method or operator is used to return the common elements from both the collections.
Here is the pictorial representation of the LINQ intersect method.
LINQ Intersect method will combine both the collections into a single collection and return only the matching element from the collection.
Syntax of LINQ Intersect Method
The syntax of using the intersect method to get the matching elements from the multiple collections.
var result = count1.Intersect(count2);
LINQ Distinct Method
In LINQ, the Distinct method or operator is used to get only the distinct elements from the collection.
Here is the pictorial representation of the LINQ Distinct method.
The LINQ Distinct method or operator is used to get only the distinct elements from the collection.
Syntax of LINQ Distinct Method
Here is the syntax of using distinct methods to get unique elements from the collection.
- string[] countries = { "UK", "India", "Australia", "uk", "india", "USA" };
- //apply the Distinct method to find out the different country names
- IEnumerable<string> result = countries.Distinct(StringComparer.OrdinalIgnoreCase);
LINQ SequenceEqual Method
In LINQ, the SequenceEqual method is used to compare the sequence of two collections that are equal or not. It determines two sequences whether they are equal or not by comparing the elements in a pair-wise manner, and two sequences contain the equality number of the element or not.
The LINQ SequenceEqual method will return the Boolean value true in case two sequence elements are equal, and all the elements match in both the sequences; otherwise, it will throw false.
Syntax of LINQ SequenceEqual Method
The syntax of using LINQ SequenceEqual method to check the given two collections are equal or not.
In the above syntax, we are using the LINQ SequenceEqual method to check whether "arr1" and "arr2" are equal or not.
string[] array1 = { "welcome", "to", "tutlane", "com" };
string[] array2 = { "welcome", "TO", "Noida", "com" };
string[] array3 = { "welcome", "to", "noida" };
string[] array4 = { "WELCOME", "TO", "NOIDA" };
//Sequence.Equal() method is used to check if both te sequences are equal or not
var res1 = array1.SequenceEqual(array2);
var res2 = array1.SequenceEqual(array2, StringComparer.OrdinalIgnoreCase);
var res3 = array1.SequenceEqual(array3);
var res4 = array3.SequenceEqual(array4, StringComparer.OrdinalIgnoreCase);
LINQ Concat Method
In LINQ, the Concat method or operator is used to concatenate or append the two collection elements into a single collection, and it does not remove the duplicates from two sequences.
Here is the pictorial representation of the LINQ Concat Method.
string[] array1 = { "a", "b", "c", "d" };
string[] array2 = { "c", "d", "e", "f" };
var result = array1.Concat(array2);
LINQ Generation Operations
In LINQ, generation operations are used to create the new sequence of the elements. In LINQ, we have a different type of generation operator methods available. These are:
- DefaultfEmpty
- Range
- Repeat
- Empty
These LINQ generation operators will help us to generate a new sequence of elements.
The following table shows more detailed information related to generation operator methods.
Method | Description |
---|---|
DefaultfEmpty | If the collection contains the empty elements, then it will return the default value. |
Empty | It returns the empty collection of sequences. |
Range | It returns the collection that contains a sequence of numbers. |
Repeat | It returns a collection that contains the one repeated value-based on a specified length. |
LINQ Range Method
In LINQ, the Range method or operator is used to generate the sequence of integer or numbers based on the specified values of the start index and end index.
Syntax of LINQ Range Method
Here is the syntax of the LINQ Range method to generate the sequence of numbers based on the specified index.
From the above syntax, we defined the Range method with two parameters. Here, the first parameter shows the starting element of the integer, and the second integer is the one which tells us the limit up to which it can display the integer in a sequence.
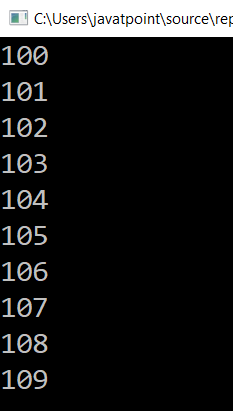
LINQ Repeat Method
In LINQ, the Repeat method or operator is used to generate the collection with the same number as the repeated times based on the specified index value.
Syntax of LINQ Repeat Method
Here is the syntax of the LINQ Repeat method to generate the repeated number based on specified index values.
In the above syntax, we defined the Repeat method with two parameters. Here the first parameter tells the starting elements of the integer, and the second parameter is the one tells how many times the same number repeated in sequence.
LINQ Empty Method
In LINQ, the Empty method or operator is used to return the empty sequence of the collection.
Syntax of LINQ Empty Method
Here is the syntax of the LINQ Empty method to generate the collection with an empty sequence
LINQ to Objects
In LINQ, if we use "LINQ to Objects" in our applications, it will give us a chance to use IEnumerable or IEnumerable<T> collections directly in LINQ queries, without the use of any intermediate LINQ provider or API such as LINQ to SQL, or LINQ to XML. By using LINQ to Objects, we can apply the query to any of the Enumerable collections such as List<T>, Array or Dictionary <TKey, TValue>.
The LINQ to Objects provides a new way to get the data from the collections with LINQ Queries, but before that, there is a need to write a lot of foreach loops to get the data from the collections.
LINQ to Objects provides more advantages when compared with a traditional foreach loop. These are:
- They provide more readability when we use them with multiple conditions.
- It enables filtering, ordering, and grouping capabilities with minimal application code.
- They are portal to any data sources with less or no modifications.
If we use LINQ in complex operations, then we will see the benefits of using the LINQ, instead of traditional iteration loops.
- LINQ to Strings
- LINQ to String Array
- LINQ to Int Array
- LINQ to Files
- LINQ to Lists
LINQ to Strings
LINQ to strings is nothing but writing the LINQ queries on String to get the required data from the string sequence. In LINQ, we can write queries on strings along with traditional string functions and regular expressions to perform the required operations on strings using the LINQ.
Syntax of LINQ to Strings
The syntax of writing the LINQ queries is:
In the above syntax, we have written the LINQ query on String to get the distinct element.
LINQ to String Array
LINQ to String Array means writing the LINQ queries on string array to get the required data. If we use LINQ queries on string arrays, we can get the required elements easily without writing the much code.
Syntax of LINQ to String Array
Here is the syntax of writing the LINQ queries on string Arrays to get the required element from array collection.
In the above syntax, we have written the LINQ query to get the data from the "arr" string array.
Expression in LINQ
The lambda Expression can be assigned to the Func or Action type delegates to process over in-memory collections. The .NET compiler converts the lambda expression assigned to Func or Action type delegate into executable code at compile time.
LINQ introduced the new type called Expression that represents strongly typed lambda expression. It means lambda expression can also be assigned to Expression<TDelegate> type. The .NET compiler converts the lambda expression which is assigned to Expression<TDelegate> into an Expression tree instead of executable code. This expression tree is used by remote LINQ query providers as a data structure to build a runtime query out of it (such as LINQ-to-SQL, EntityFramework or any other LINQ query provider that implements IQueryable<T> interface).
The following figure illustrates differences when the lambda expression assigned to the Func or Action delegate and the Expression in LINQ.

We will learn Expression tree in the next section but first, let's see how to define and invoke an Expression.
Define an Expression
Take the reference of System.Linq.Expressions namespace and use an Expression<TDelegate> class to define an Expression. Expression<TDelegate> requires delegate type Func or Action.
For example, you can assign lambda expression to the isTeenAger variable of Func type delegate, as shown below:
public class Student
{
public int StudentID { get; set; }
public string StudentName { get; set; }
public int Age { get; set; }
}
Func<Student, bool> isTeenAger = s => s.Age > 12 && s.Age < 20;
Expression<Func<Student, bool>> isTeenAgerExpr = s => s.Age > 12 && s.Age < 20;
//compile Expression using Compile method to invoke it as Delegate
Func<Student, bool> isTeenAger = isTeenAgerExpr.Compile();
//Invoke
bool result = isTeenAger(new Student() {Name = "Steve", Age = 20 });
Console.WriteLine(result);
Comments
Post a Comment