.Net Core
User Secrets
Acton filter
To create an Acton filter, we need to create a class that inherits either from the
IActionFilter
or IAsyncActionFilter
To implement the synchronous Action filter that runs before and after action method execution, we need to implement
OnActionExecuting
OnActionExecuted methods:
namespace ActionFilters.Filters
{
public class ActionFilterExample : IActionFilter
{
public void OnActionExecuting(ActionExecutingContext context)
{
// our code before action executes
}
public void OnActionExecuted(ActionExecutedContext context)
{
// our code after action executes
}
}
}
We can do the same thing with an asynchronous filter by inheriting from IAsyncActionFilter, but we only have one method to implement the OnActionExecutionAsync:
namespace ActionFilters.Filters
{
public class AsyncActionFilterExample : IAsyncActionFilter
{
public async Task OnActionExecutionAsync(ActionExecutingContext context, ActionExecutionDelegate next)
{
// execute any code before the action executes
var result = await next();
// execute any code after the action executes
}
}
}
GLOBAL FILTER
If we want to use our filter globally, we need to register it inside the AddMvc() method in the ConfigureServices method:
services.AddMvc(
config =>
{
config.Filters.Add(new GlobalFilterExample());
});
But if we want to use our filter as a service type on the Action or Controller level, we need to register it in the same ConfigureServices method but as a service in the IoC container:
services.AddScoped<ActionFilterExample>();
services.AddScoped<ControllerFilterExample>();
What's the difference between .NET Core, .NET Framework
-
Cross-Platform needs
-
Best performant and scalable systems
-
Command line style development for Mac, Linux or Windows
- NET core as an open source
- Easy to build cross-platform asp.net app on Windows, Mac, and Linux.
- It runs on .Net Core and Full .Net Framework.
- Asp.Net Core does not support WebForm. It supports MVC, Web API and Asp.Net Web pages originally added in .Net Core 2.0.
- Core did not support Web.config and Global.asax files. It is supporting appsettings.json.
- Core Browser refresh will compile and executed the code no need for re-compile.
- Ability to host on:
- A) Kestral
- B) IIS
- C) Apache
- E) Docker
Inbuilt Dependency Injection (DI) support for ASP.NET Core
ASP.NET Core applications, dependency injection is inbuilt i.e. no setup headache for DI. Just create some services and get ready to use DI sample Core MVC application has DI inbuilt in it, let’s open “Startup.cs” and look for“ConfigureServices(IServiceCollection services)” method Its main purpose is the configuration of services like EF, Authentication, adding MVC handwritten custom services like IEmailServer and ISmsSender or custom service
User Secrets of ASP.NET Core
What is a Kestrel Web Server?
https://www.youtube.com/watch?v=3RpZGZDMd2A
As we already discussed the ASP.NET Core is a cross-platform framework. It means it supports developed and run applications on different types of operating systems such as Windows, Linux, or Mac.
The Kestrel is the cross-platform web server for the ASP.NET Core application. That means this Server supports all the platforms and versions that the ASP.NET Core supports. By default, it is included as the internal web server in the .NET Core application.
As we already discussed the ASP.NET Core is a cross-platform framework. It means it supports developed and run applications on different types of operating systems such as Windows, Linux, or Mac.
The Kestrel is the cross-platform web server for the ASP.NET Core application. That means this Server supports all the platforms and versions that the ASP.NET Core supports. By default, it is included as the internal web server in the .NET Core application.
NET Core CLI
- When we run an ASP.NET Core application using the .NET Core CLI, then the .NET Core runtime uses Kestrel as the webserver
- The .NET Core CLI (Command Line Interface) is a cross-platform tool that is used for developing ASP.NET core applications on cross-platform such as windows, macs or Linux
Open command prompt and type “dotnet —” and press enter as shown below.
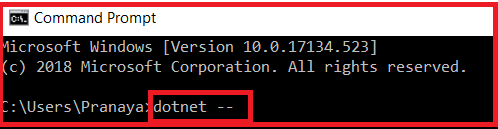
Once you type the “dotnet —” and click on the enter button then you will find lots of commands as shown below.
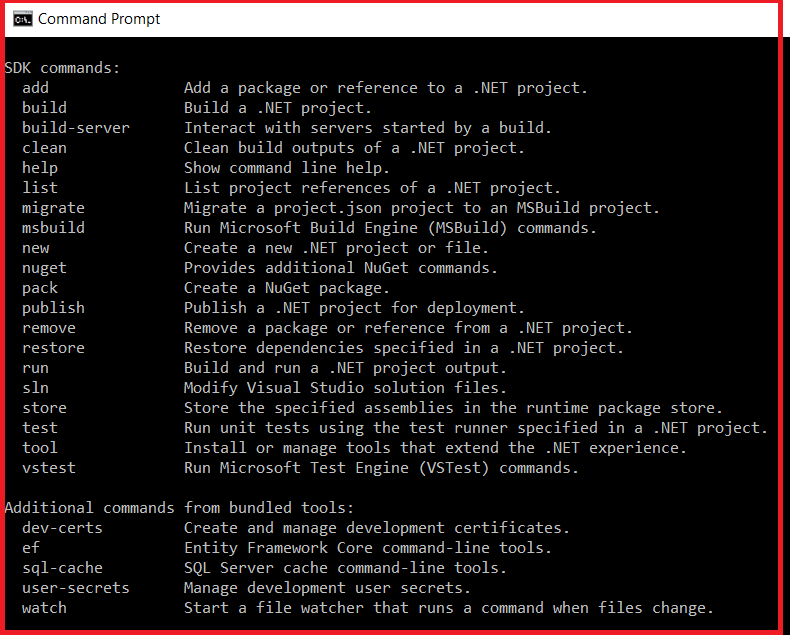
Using the CLI (above commands)
- You can create a new project using the new command, you can also build the project using the build command, or you can publish the project using the publish command.
- It is possible to restore the dependencies and tools which are required for a .net core project using the CLI
Now, let’s examine the project structure:
In ASP.NET Core, Dependencies is the place where the necessary dll.s for the application are stored.
Under the Properties menu, you will see a file called launchSettings.json which describes how a project can be launched. It describes the command to run, whether the browser should be opened, which environment variables should be set, and so on.

Static content is hosted in the wwwroot folder. The content such as CSS, Javascript files and Bootstrap, jquery libraries need to be included here.
Controller, Models and Views folders are automatically created as we chose Web Application (Model-View-Controller) in the above screenshot. These support MVC pattern and we will add new files to these folders in the following posts while building web applications.
appsettings.json is used to store information such as connection strings or application specific settings and these are stored in the JSON format as the file extension suggests. (If you are familiar with ASP.NET MVC you may notice that the function of this file is similar to Web.config)

At the bottom there are two classes: Program.cs and Startup.cs.
Program.cs is the main entry point for the application. It then goes to the Startup.cs class to finalize the configuration of the application.

Startup.cs includes Configure and ConfigureServices methods.

The Dependency Injection pattern is used heavily in ASP.NET Core architecture. It includes built-in IoC container to provide dependent objects using constructors.
The
ConfigureServices
method is a place where you can register your dependent classes with the built-in IoC container (ASP.NET Core refers dependent class as a Service). After registering the dependent class, it can be used anywhere in the application. You just need to include it in the parameter of the constructor of a class where you want to use it. The IoC container will inject it automatically.
The
Configure
method is used to specify how the app responds to HTTP requests. The request pipeline is configured by adding middleware components to an IApplicationBuilder
instance. IApplicationBuilder
is available to the Configure
method, but it isn’t registered in the service container. Hosting creates an IApplicationBuilder
and passes it directly to the Configure
method.
The default route for the MVC application is the
Index
method in the Home controller as specified below in the Configure
method:
Index
method in the HomeController.cs
returns the view Index.cshtml
which can be found under /Views/Home/ .
The execution sequence of the application is as follows:

Comments
Post a Comment