Project & Notes-2 - ASP.NET CORE SYLLABUS V2
C:\Users\vasan\OneDrive\Desktop\Online Class\MVC Class Projects\ValidationDataAnnotation
Projects - C:\Users\vasan\OneDrive\Desktop\Online Class\MVC Class Projects
- URL helpers Server-side Data Receiving Ways
Action Parameters
View Models/Objects
FormCollection
https://dotnettutorials.net/lesson/formcollection-mvc/
What is the FormCollection Class in ASP.NET MVC?
The FormCollection class in ASP.NET MVC will automatically receive the posted form values in the controller action method in the form of key/value pairs. The values can be accessed using either key names or indexes. We can use the FormCollection to loop through each key and its value that is posted to the server. Let’s add the following Create Post method in the employee Controller class.
Reusable UI Components
Creating ViewModel
Understanding ASP.NET Core MVC Validation
C:\Users\vasan\OneDrive\Desktop\Online Class\MVC Class Projects\ValidationDataAnnotation
The model is the M in MVC. The data model represents the core business data/information that an application is being used to access and manipulate. The model is the center of any application. The model encapsulates the storage and validation concerns. HTML helpers are provided by ASP.NET MVC to do the input validations. Additionally, we can also use data annotation attributes from the namespace to do validations at the model level. Data annotation attributes are attached to the properties of the model class and enforce some validation criteria. They are capable of performing validation on the server side as well as on the client side
Some frequently used tags are,
- Required
- Regular Expression
- Range
- EmailAddress
- DisplayName
- DataType
- StringLength
- UIHint
- DataType
- StringLength
- MaxLength
- MinLength
Need of Server Side and Client Side Validation
Validation with Data Annotation
Custom Server and Client side validation
client - data annotation
DataAnnotations is used to configure your model classes, which will highlight the most commonly needed configurations. DataAnnotations are also understood by a number of .NET applications, such as ASP.NET MVC, which allows these applications to leverage the same annotations for client-side validations
Server side - Inside action method (ex. checking text box value ,etc)
Data Passing Techniques
ViewData, ViewBag and TempData
Session
Query String
What is the query string?
URL's are made up of several parts, like protocol, hostname, path and so on. The query string is the part of the URL that comes after a question-mark character. So, in a URL like this:
https://www.google.com/search?q=test&oq=hello
Everything after the ? character is considered the query string. In this case, there are two parameters: One called q and one called oq. They have the values "test" and "hello". These would be relevant to the page displayed by the URL - for instance, the q parameter is likely used to tell the server what the user just searched for. The query string is usually appended as the result of the user clicking on a link or submitting a FORM. This allows the same file on your server to handle multiple situations - it can vary the output returned based on the input through the query string.
Accessing the query string
Access to the query string in ASP.NET MVC is very simple - just use the HttpContext class, which this entire chapter is about. The information about the query string is located using one of the following properties: HttpContext.Request.QueryString and HttpContext.Request.Query. The difference is that the QueryString is basically the raw text string, while the Query property allows you to easily access keys and their values
Cookies
Cookie Vs. Session
|
|
|
|
|
|
LINQ Fundamentals
* LinQ is a 'Language Integrated Query
* LINQ provides the new way to manipulate the data, whether it is to or from the database or with an XML file or with a simple list of dynamic data. LINQ is a uniform query system in C# to retrieve the data from different sources of data and formats
* LINQ is built in C#. It is used to retrieve the data from different types of sources such as XML, docs, collections, ADO.Net DataSet, Web Service, MS SQL Server, and other databases server.
LINQ has three ways to write the queries:
- Using Query Syntax
- Using Method Syntax
- Using Mixed Syntax
Many times we use a combination of both. Now, with the evolution of the entity framework, the importance of LINQ has grown much higher as compared to old times.
have different types of LINQ Objects available in C# and VB.NET.
- LINQ To Objects
- LINQ To DataSets
- LINQ To SQL
- LINQ to XML
- LINQ To Entities
Advantages of LINQ
In our applications, the benefits of LINQ are:
- We do not need to learn new query language syntaxes for different sources of data because it provides the standard query syntax for the various data sources.
- In LINQ, we have to write the Less code in comparison to the traditional approach. With the use of LINQ, we can minimize the code.
- LINQ provides the compile-time error checking as well as intelligence support in Visual Studio. This powerful feature helps us to avoid run-time errors.
- LINQ provides a lot of built-in methods that we can be used to perform the different operations such as filtering, ordering, grouping, etc. which makes our work easy.
- The query of LINQ can be reused.
Disadvantages of LINQ
Disadvantages of LINQ are:
- With the use of LINQ, it's very difficult to write a complex query like SQL.
- It was written in the code, and we cannot make use of the Cache Execution plan, which is the SQL feature as we do in the stored procedure.
- If the query is not written correctly, then the performance will be degraded.
- If we make some changes to our queries, then we need to recompile the application and need to redeploy the dll to the server.
LINQ Query Syntax
LINQ is one of the easiest ways to write the complex LINQ Queries in an accessible and readable format. The syntax of this type of Query is much similar to SQL queries.
Syntax of LINQ is as:
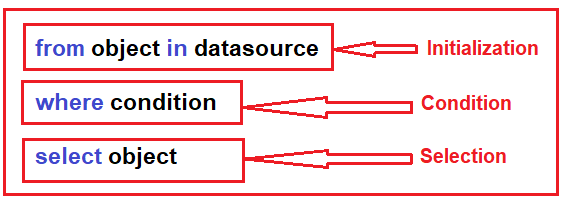
LINQ Query Syntax in C#
int[] Num = { 1, 2, 3, 4, 5, 6, 7, 8, 9 };
IEnumerable<int> result = from numbers in Num
where numbers >3
select numbers;
LINQ Method Syntax
Method Syntax becomes the most popular nowadays to write LINQ queries. It uses a lambda expression to define the condition for the Query. Method Syntax is easy to write simple queries to perform the read-write operations on a particular data source. For the complex queries, Method Syntaxes are a little hard as compared to the Query Syntax.
- List<int>integerList = new List<int>()
- {
- 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
- };
- //LINQ Query using Method Syntax
- varMethodSyntax = integerList.Where(obj =>obj> 5).ToList();
- //Execution
- foreach (var item inMethodSyntax)
- {
- Console.Write(item + " ");
- }
LINQ Mixed Syntax
LINQ Mixed Syntax is a combination of both Query and MethodSyntax.
- List<int>integerList = new List<int>()
- {
- 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
- };
- //LINQ Query using Mixed Syntax
- varMethodSyntax = (fromobjinintegerList
- whereobj> 5
- selectobj).Sum();
- //Execution
- Console.Write("Sum Is : " + MethodSyntax);
This whole syntax forms a Lambda Expression.
Here, we are taking an example of commonly used Expression:
X=>x+10
- //list to store the countries type of string
- List<string> countries = new List<string>();
- countries.Add("India");
- countries.Add("US");
- countries.Add("Australia");
- countries.Add("Russia");
- //use lambda expression to show the list of the countries
- IEnumerable<string> result = countries.Select(x => x);
- //foreach loop to display the countries
- foreach (var item in the result)
- {
- Console.WriteLine(item);
- }
LINQ Element Operators
In LINQ, element operators are used to returning the first and last element of the list or single element from the collection or a specific element based on the index value from the collection.
In LINQ, we have different types of element operators available. These are:
- First
- FirstOrDefault
- Last
- LastOrDefault
- ElementAt
- ElementAtOrDefault
- Single
- SingleOrDefualt
- DefaultfEmpty
Operators | Description |
---|---|
First | It returns the first element in sequence or from the collection based on the condition. |
FirstOrDefault | It is the same as First, but it returns the default value in case when no element is found in the collection. |
Last | It returns the last element in sequence or the last element based on the matching criteria. |
ElementAt | It returns an element from the list based on the specific index position. |
ElementAtOrDefault | It is the same as ElementAt, but it returns the default value in case if no element is present at the specified index of the collection. |
Single | It returns the single specific element from the collection. |
SingleOrDefault | It is the same as Single, but it returns the default value in case if no element is found in the collection. |
DefaultfEmpty | It returns the default value in case if the list or collection contains empty or null values. |
LINQ First() Element
In LINQ, the First () method/operator is used to return the first element from the sequence of the items in the list or collection or the first element in the sequence of items in the list based on the specified condition. In case if no element is present in the list/collection based on the specified condition, then LINQ FIRST () method will throw an error.
If there is no values it will throw an error.
Syntax of LINQ FIRST () method
The syntax of the first method to get the first element from the list is:
From the above syntax, we are getting the first element from the "objList" collection using the LINQ FIRST () method.
- int[] objList = { 1, 2, 3, 4, 5 };
- //First() method is used to return the first element from the array 'objList'
- int result = objList.First();
- Console.WriteLine("Element from the List: {0}", result);

LINQ FirstOrDefault() Method
In LINQ, FirstOrDefault() Operator is used to return the first element from the list/collection. FirstOrDefault() Operator is same as LINQ First() Operator and the only difference is, in case if the lists returns no elements then LINQ FirstOrDefault operator method will return default value.
Syntax of FirstOrDefault Method
Here is the syntax of the LINQ FirstOrDefault operator to return the first element from the list or in case if lists return no element.
From the above syntax, we are trying to get the First element or default element from the "objList" collection by using LINQ FirstOrDefault() operator.
LINQ Last() Method
The last () Method in LINQ is used to return the last element from the list/collection. LINQ Last() method will throw an exception in case if the list/collection returns no elements.
Syntax of LINQ Last() method
LINQ LastOrDefault() Method
In LINQ, LastOrDfeault() method/operator is used to return the last element from the list/collection, and it is the same as LINQ Last() method, and the only difference is that it will return the default value when there is no element in the list/collection.
Syntax of LINQ LastOrDefault() Method
Here is the syntax of using the LINQ LastOrDefault() method to get the last element from the list or default value. In case, if lists returns no elements using the LINQ LastOrDefault() method.
From the above syntax, we are trying to get the last element from the "LastObj" list using LINQ LastOrDefault() method.
LINQ ElementAt() Method
In LINQ, ElementAt() operator is used to return the elements from the list/collection based on the specified index.
Generally, in the list, the index will start with zero, so if we want to get the first element, then there is a need to send zero (0) as an index position.
Syntax of LINQ ElementAt() Method
LINQ ElementAtOrDefault() Method
In LINQ, ElementAtOrDefault() method is used to get the element at specified index position in the list/collection and it is same as LINQ ElementAt() method. The only difference between the both ElementAtOrDefault() and ElementAt() is that it will return default value only. On the other hand, if the specified index position of the element does not exist in the list, then in that case also this will return the default value.
Syntax of LINQ ElementAtOrDefault() Method
The syntax of using the LINQ ElementAtOrDefault() to get the element at specified index position.
LINQ Single() Method
In LINQ, the Single() method is used to return the single element from the collection, which satisfies the condition. In case, if the Single() method found more than one element in collection or no element in the collection, then it will throw the "InvalidOperationException" error.
Syntax of LINQ Single () Method
The syntax of using the LINQ Single () method to get a single element from the collection.
- List<Student> objStudent = new List<Student>()
- {
- new Student() { Name = "Shubham Rastogi", Gender = "Male",Location="Chennai" },
- new Student() { Name = "Rohini Tyagi", Gender = "Female", Location="Chennai" },
- new Student() { Name = "Praveen Alavala", Gender = "Male",Location="Bangalore" },
- new Student() { Name = "Sateesh Rastogi", Gender = "Male", Location ="Vizag"},
- new Student() { Name = "Madhav Sai", Gender = "Male", Location="Nagpur"}
- };
- //initialize the array objList
- int[] objList = { 1 };
- //objStudent.Single() used to select the student
- var user = objStudent.Single(s => s.Name == "Shubham Rastogi");
- string result = user.Name;
- int val = objList.Single();
- Console.WriteLine("Element from objStudent: {0}", result);
- Console.WriteLine("Element from objList: {0}", val);
LINQ SingleOrDefault Method
In LINQ, SingleOrDefault() method is used to return the single element. In case if there are no elements present in the list/collection, then this will return more than one element and will throw an exception like a Single () method.
Syntax of LINQ SingleOrDefault() Method
Here is the syntax of using the LINQ SingleOrDefault() method to get the single element from the collection.
If the number object has more than one value it'll throw an error
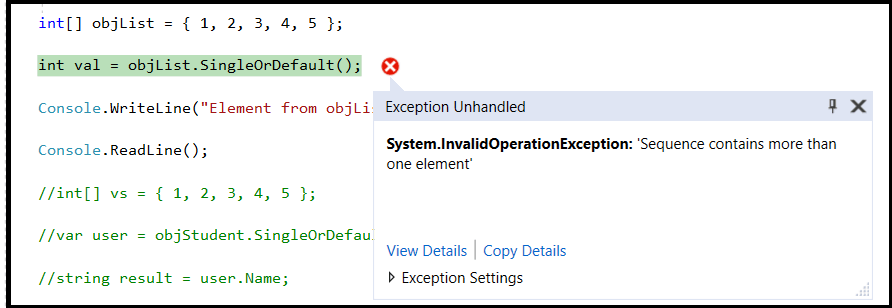
LINQ DefaultfEmpty() Method
In LINQ, DefaultfEmpty() method is used to return the default value in that case if the list/collection contains the null or empty value; otherwise, it will return the element from the sequence in the collection.
Syntax of using the LINQ DefaultfEmpty() method to get the list of the elements when the list returns an empty or null value.
- //create an array 'b'
- int[] b = { };
- int[] a = { 1, 2, 3, 4, 5 };
- //with the help of DefaultfEmpty try to fetch the value from both of the list
- var result = a.DefaultIfEmpty();
- var result1 = b.DefaultIfEmpty();
- Console.WriteLine("----List1 with Values----");
- //with the help of foreach loop we will print the value of 'result' variable
- foreach (var val1 in result)
- {
- Console.WriteLine(val1);
- }
- Console.WriteLine("---List2 without Values---");
- //with the help of foreach loop we will print the value of 'result1' variable
- foreach (var val2 in result1)
- {
- Console.WriteLine(val2);
- }
Output:
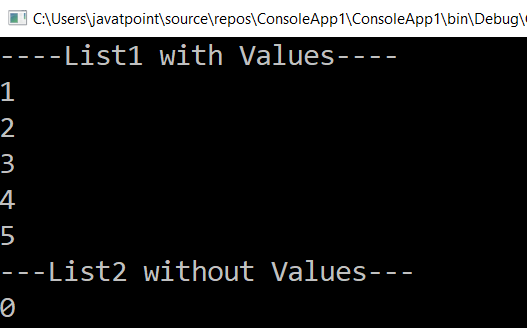
LINQ Join() Operators
In LINQ, Join() operators are used to join the two or more lists/collections and get the matched data from the collection based on the specified conditions. The behavior and functionality of Join() operators are the same as SQL joins.
In LINQ, We have different types of joins available. These are:
- INNER JOIN
- LEFT OUTER JOIN
- CROSS JOIN
- GROUP JOIN
Operator | Description |
---|---|
Inner Join | It returns the element from the collections which satisfy the specified expression. |
Left Outer Join | It returns all the elements from the left side collection and matching the elements with the right side collection. |
Cross Join | It returns the Cartesian product of elements in collections. |
Group Join | The join clause with an 'into' expression is called a group join |
LINQ Inner Join
In LINQ, Inner join is used to return only the matched records or elements from the collections based on the specified conditions.
Syntax of LINQ Inner Join
Here is the syntax of using the LINQ Inner join to get the elements from the collections based on the specified condition.
- List<Department> Dept = new List<Department>
- (){
- new Department{DepId=1,DepName="Software"},
- new Department{DepId=2,DepName="Finance"},
- new Department{DepId=3,DepName="Health"}
- };
- List<Employee>Emp = new List<Employee>
- ()
- {
- new Employee { EmpId=1,Name = "Akshay Tyagi", DeptId=1 },
- new Employee { EmpId=2,Name = "Vaishali Tyagi", DeptId=1 },
- new Employee { EmpId=3,Name = "Arpita Rai", DeptId=2 },
- new Employee { EmpId=4,Name = "Sateesh Alavala", DeptId =2},
- new Employee { EmpId=5,Name = "Madhav Sai"}
- };
- var result = from d in Dept
- join e in Emp
- on d.DepId equals e.DeptId
- select new
- {
- EmployeeName = e.Name,
- DepartmentName = d.DepName
- };
- foreach (var item in result)
- {
- Console.WriteLine(item.EmployeeName + "\t | " + item.DepartmentName);
- }
LINQ Left Outer Join
In LINQ, LEFT JOIN or LEFT OUTER JOIN is used to return all the records or elements from the left side collection and matching the elements from the right side of the collection.
In LINQ, to achieve the LEFT Join behavior, it is mandatory to use the "INTO" keyword and "DefaultfEmpty()" method.
Syntax of LINQ Left Outer Join
The syntax of using the LINQ Left Outer Join to get all the elements from the collection and matching the element from the right collection.
LINQ Cross Join
In LINQ, Cross join will produce the Cartesian product of the collections of items. There is no need for any condition to join the collection.
In LINQ Cross join, each element on the left side collection will be mapped to all the elements on the right side collection.
Syntax of LINQ Cross Join
Here is the syntax of using the LINQ Cross join to get the Cartesian product of the collection items.
LINQ Group Join
In LINQ, a Join clause with an 'into' expression is called a Group join. In LINQ, Group join produces a sequence of the elements of the objects, which is based on the matching element from both left and right collections.
In case, if no matching element found from the right collection with the left collection, then the join clause will return an empty array. In LINQ, Group join is an equivalent to inner equi join except for the result of the elements organized into groups.
Syntax of LINQ Group Join
The syntax of using the LINQ Group Join to get the matching element from the given collections based on our requirement.
From the above syntax, we used a Join clause with an 'into' expression to get the matching elements from the collections.
Deferred Execution vs. Immediate Execution
SQL Joins with LINQ
Lazy Loading vs. Eager Loading
LINQPad
https://www.c-sharpcorner.com/UploadFile/97fc7a/generate-linq-sql-queries-with-linqpad/
https://www.linqpad.net/Download.aspx
Understanding and Configuring LINQPad
LINQPad Querying SQL Server database LINQPad Querying DAL layer DLL using
Well, DAL stands for Data Access Layer and BLL, stands for Business Logic Layer.
Introduction to Entity Framework Core ORMs used with .NET
EF6 vs. EF Core
Advantages of Entity Framework
Database Migration, DB Procedures and Functions Entity Framework Code First Migrations
Updating Database when the Model Changes
Calling Stored Procedures and functions
Code First with existing Database
Repository Design Pattern and Unit of Work Design Patterns
Understanding Repository and UOW Design Pattern Need of Repository Design Pattern
Need to Unit of Work Design Pattern
Implementing Repository and UOF Design Pattern Dependency Injection
Understanding Dependency Injection
Need of Dependency Injection
Implementing DI
ASP.NET Core MVC Authentication: Identity ASP.NET Core MVC Authentication Options Introduction to Identity
Implementing Identity
ASP.NET Core MVC Pipeline, Middleware and Filters Exploring ASP.NET Core Pipeline
ASP.NET Core MVC Middleware
ASP.NET Core MVC Filters
Extending ASP.NET Core MVC Filters
Configuring ASP.NET Core MVC Filters
Securing ASP.NET Core MVC App
Implementing Authorization using Authorization Filter Passing Logged in User Info Across the App
- Get link
- X
- Other Apps
Comments
Post a Comment