Asp.net Trainning
Demo Sql & session , redirect etc - C:\Users\vasan\OneDrive\Desktop\Online Class\Demo
Web service -C:\Users\vasan\OneDrive\Desktop\Online Class\Projects\Web service\WebApplication3
IFrame & Application object - C:\Users\vasan\OneDrive\Desktop\Online Class\rajesh\deleteproj\deleteproj
c# - https://www.tutorialspoint.com/asp.net/asp.net_custom_controls.htm
page life cycle - https://www.c-sharpcorner.com/UploadFile/8911c4/page-life-cycle-with-examples-in-Asp-Net/
net_validators - https://www.tutorialspoint.com/asp.net/asp.net_validators.htm
ex - n.workinig - C:\Users\vasan\OneDrive\Desktop\Online Class\rajesh\TestProject\TestProject
Validations - https://drive.google.com/file/d/1bsqpCjQc1R-001XKIKIb0HqPPoCrVWRy/view
State , cookie , session, redirect C:\Users\vasan\source\repos\Insert_view_Asp_net\Insert_view_Asp_net
A Repeater is a Data-bound control. Data-bound controls are container controls. It creates a link between the Data Source and the presentation UI to display the data. The repeater control is used to display a repeated list of items.
A Repeater has five inline templates to format it:
- <HeaderTemplate> - Displays Header text for a Data Source collection and applies a different style for the Header text.
- <AlternatingItemTemplate> - Changes the background color or style of alternating items in a Data Source collection.
- <Itemtemplate> - It defines how the each item is rendered from the Data Source collection.
- <SeperatorTemplate> - It will determine the separator element that separates each item in the item collection. It will be a <br> or <Hr> HTML element.
- <FooterTemplate> - Displays a footer element for the Data Source collection.
Overview of ADO.NET
The .NET Framework includes its own data access technology i.e. ADO.NET. ADO.NET is the latest implementation of Microsoft’s Universal Data Access strategy. ADO.NET consists of managed classes that allows .NET applications to connect to data sources such as Microsoft SQL Server, Microsoft Access, Oracle, XML, etc., execute commands and manage disconnected data..t
Microsoft ADO.NET is the latest improvement after ADO. Firstly, ADO.NET was introduced in the 10th version of the .NET framework, which helps in providing an extensive array of various features to handle data in different modes, such as connected mode and disconnected mode. In connected mode, we are dealing with live data and in disconnected mode, data is provided from the data store.
ADO.NET was primarily developed to address two ways to work with data that we are getting from data sources. The two ways are as follows :
- The first is to do with the user’s need to access data once and to iterate through a collection of data in a single instance.
- The second way to work with data is disconnected architecture mode, in which we have to grab a collection of data and we use this data separately from the data store itself.
Architecture of ADO.NET :
ADO.NET uses a multilayered architecture that revolves around a few key concepts as –
- asConnection
- Command
- DataSet objects
The ADO.NET architecture is a little bit different from the ADO, which can be shown from the following figure of the architecture of ADO.NET.
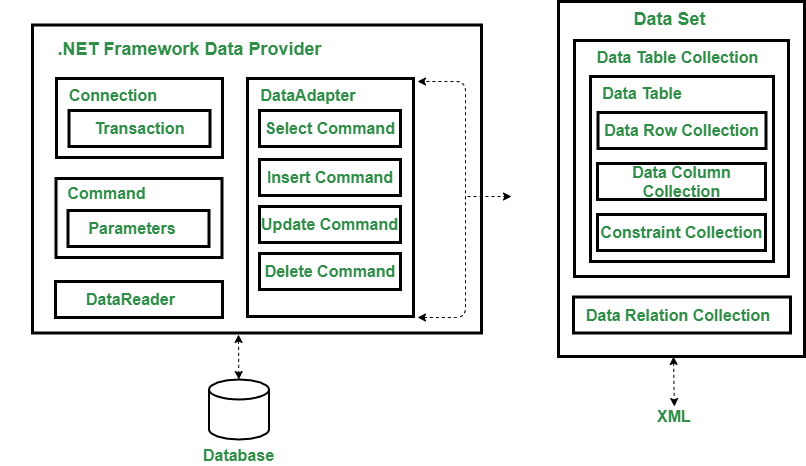
Architecture of ADO.NET
Features of ADO.NET :
The following are the features of ADO.NET –
- Interoperability-
We know that XML documents are text-based formats. So, one can edit and edit XML documents using standard text-editing tools. ADO.NET uses XML in all data exchanges and for internal representation of data. - Maintainability –
ADO.NET is built around the idea of separation of data logic and user interface. It means that we can create our application in independent layers. - Programmability (Typed Programming) –
It is a programming style in which user words are used to construct statements or evaluate expressions. For example: If we want to select the “Marks” column from “Kawal” from the “Student” table, the following is the way to do so:
DataSet.Student("Kawal").Marks;
- Performance –
It uses disconnected data architecture which is easy to scale as it reduces the load on the database. Everything is handled on the client-side, so it improves performance. - Scalability –
It means meeting the needs of the growing number of clients, which degrading performance. As it uses disconnected data access, applications do not retain database lock connections for a longer time. Thus, it accommodates scalability by encouraging programmers to conserve limited resources and allow users to access data simultaneously Connected V/S Disconnected Architecture In C#
In C#, we work in two different ways with database connectivity. As you know when we create software or website or any other application which is connected to the database we need to make connection to the database to get data. Here you can get data in two different ways.
Either it can be connected architecture where you go and connect to the database and get data or disconnected architecture where you connect to the database first time and get all data in an object and use it if required. You can perform any action like insert, update, and search on this.
You can use two C# objects to achieve this, first one is DataSet and other one is DataReader.
DataSet
It is a type of disconnected architecture. Disconnected architecture means, you don’t need to connect always when you want to get data from the database. You can get data from dataset; basically DataSet is a collection of datatables. We can store the database table, view data in the DataSet and can also store the xml value in dataset and get it if required.
To achieve this you need to use DataAdapter which work as a mediator between Database and DataSet.
Example
- public DataSet GetEmployeeData()
- {
- SqlConnection conString = new SqlConnection("myconnection");
- conString.Open();
- SqlCommand cmdQuery = new SqlCommand("Select * from Employee", conString);
- SqlDataAdapter sda = new SqlDataAdapter(cmdQuery);
- DataSet dsData = new DataSet();
- sda.Fill(dsData);
- return dsData;
- }
It is a connected architecture, which means when you require data from the database you need to connect with database and fetch the data from there. You can use if you need updated data from the database in a faster manner. DataReader is Read/Forward only that means we can only get the data using this but we cannot update or insert the data into the database. It fetches the record one by one.
Example
- static void HasRows(SqlConnection connection)
- {
- using (connection)
- {
- SqlCommand command = new SqlCommand("SELECT CategoryID, CategoryName FROM Categories;",connection);
- connection.Open();
- SqlDataReader reader = command.ExecuteReader();
- if (reader.HasRows)
- {
- while (reader.Read())
- {
- Console.WriteLine("{0}\t{1}", reader.GetInt32(0),reader.GetString(1));
- }
- }
- else
- {
- Console.WriteLine("No rows found.");
- }
- reader.Close();
- }
- }

ADO.NET SQL Server Connection
You can connect your VB.Net application to data in a SQL Server database using the Microsoft .NET Framework Data Provider for SQL Server. The first step in a VB.Net application is to create an instance of the Server object and to establish its connection to an instance of SQL Server.
The SqlConnection Object is Handling the part of physical communication between the application and the SQL Server Database. An instance of the SqlConnection class in .NET Framework is supported the Data Provider for SQL Server Database. The SqlConnection instance takes Connection String as argument and pass the value to the Constructor statement. When the connection is established , SQL Commands may be executed, with the help of the Connection Object, to retrieve or manipulate data in the database. Once the Database activities over , Connection should be closed and release the database resources .
Sql Server connection string
If you have a named instance of SQL Server, you'll need to add that as well.
The Close() method in SqlConnection class is used to close the Database Connection. The Close method rolls back any pending transactions and releases the Connection from the SQL Server Database
Command Object
- The Command Object uses the connection object to execute SQL queries.
- The queries can be in the Form of Inline text, Stored Procedures or direct Table access.
- An important feature of Command object is that it can be used to execute queries and Stored Procedures with Parameters.
- If a select query is issued, the result set it returns is usually stored in either a DataSet or a DataReader object.
Property | Type of Access | Description |
Connection | Read/Write | The SqlConnection object that is used by the command object to execute SQL queries or Stored Procedure. |
CommandText | Read/Write | Represents the T-SQL Statement or the name of the Stored Procedure. |
CommandType | Read/Write | This property indicates how the CommandText property should be interpreted. The possible values are:
|
CommandTimeout | Read/Write | This property indicates the time to wait when executing a particular command. Default Time for Execution of Command is 30 Seconds. The Command is aborted after it times out and an exception is thrown. |
Property | Description |
ExecuteNonQuery | This method executes the command specifies and returns the number of rows affected. |
ExecuteReader | The ExecuteReader method executes the command specified and returns an instance of SqlDataReader class. |
ExecuteScalar | This method executes the command specified and returns the first column of first row of the result set. The remaining rows and column are ignored. |
ExecuteXMLReader | This method executes the command specified and returns an instance of XmlReader class. This method can be used to return the result set in the form of an XML document |
ADO.NET DataAdapter
The DataAdapter works as a bridge between a DataSet and a data source to retrieve data. DataAdapter is a class that represents a set of SQL commands and a database connection. It can be used to fill the DataSet and update the data source
DataReader in ADO.NET
An Easy Walk through the Data

Initializing DataReader
- SqlCommand cmd = new SqlCommand(SQL, conn);
- // Call ExecuteReader to return a DataReader
- SqlDataReader reader = cmd.ExecuteReader();
# ADO.NET DataReader
DataReader Object in ADO.NET is a stream-based , forward-only, read-only retrieval of query results from the Data Sources , which do not update the data. The DataReader cannot be created directly from code, they can created only by calling the ExecuteReader method of a Command Object.
The DataReader Object provides a connection oriented data access to the Data Sources. A Connection Object can contain only one DataReader at a time and the connection in the DataReader remains open, also it cannot be used for any other purpose while data is being accessed. When we started to read from a DataReader it should always be open and positioned prior to the first record. The Read() method in the DataReader is used to read the rows from DataReader and it always moves forward to a new valid row, if any row exist .
Usually we are using two types of DataReader in ADO.NET. They are SqlDataReader and the OleDbDataReader . The System.Data.SqlClient and System.Data.OleDb are containing these DataReaders respectively. From the following link you can see in details about these classes in C#.
Comments
Post a Comment